CPT Progress Blog
Current Assignments:
- Cards Game
JSON/jQuery Notes for later
General
-
First, you’ll need to create an HTML table on your website where you want to display the scores. You can do this using standard HTML table elements, such as
<table>
,<tr>
, and<td>
. -
Next, you’ll need to retrieve the highest scores data from your backend system. This data is usually stored in a database, and you can use a server-side language (e.g., PHP, Python, Ruby) to query the database and retrieve the data. The server-side script should then encode the data in JSON format and return it to the client (your website).
-
Once the data is returned to the client, you can use jQuery to parse the JSON data and display it on the HTML table. You can do this by making an AJAX (Asynchronous JavaScript and XML) request to the server-side script and using the $.getJSON method to retrieve the JSON data.
-
Finally, you can use jQuery to append the data to the appropriate cells in the HTML table. You can use the .append() method to add the data as a new child element to the table cells. Method 1:
Step 3
Method 1
To retrieve the JSON data from the server-side script, you can use an AJAX request. AJAX allows you to make requests to the server and update a specific part of the webpage without reloading the entire page.
Here’s an example of how you can make an AJAX request using jQuery:
$.ajax({
url: 'server-side-script.php',
dataType: 'json',
success: function(data) {
// data contains the JSON data returned by the server
}
});
Method 2
You can also use the $.getJSON method, which is a shorthand for making an AJAX request and expecting a JSON response:
$.getJSON('server-side-script.php', function(data) {
// data contains the JSON data returned by the server
});
In both examples, the server-side-script.php file is the server-side script that retrieves the data from the database and returns it in JSON format.
Step 4
Once you have the JSON data, you can use jQuery to append it to the HTML table. Here’s an example of how you can do this:
$.getJSON('server-side-script.php', function(data) {
// Iterate through the data and append it to the table
$.each(data, function(index, item) {
$('#table-body').append(
'<tr>' +
'<td>' + item.name + '</td>' +
'<td>' + item.score + '</td>' +
'</tr>'
);
});
});
In this example, #table-body
is the ID of the <tbody>
element in the HTML table, and item.name
and item.score
are the name and score of the player, respectively.
Game Research
Logos: Github, Stack Overflow, Discord, VS Code, Amazon Servers, Fastpages, Python, HTML
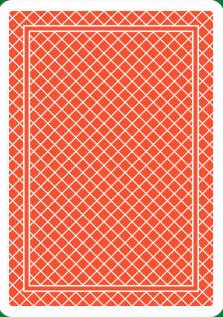
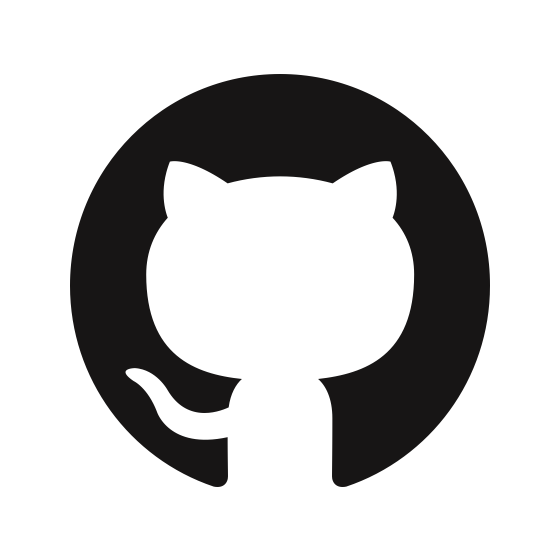
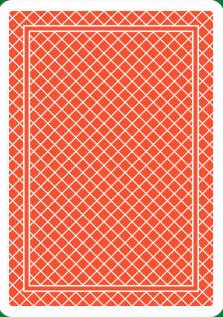

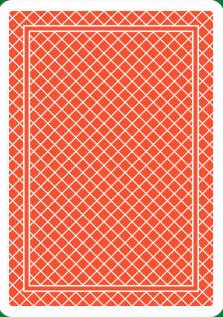

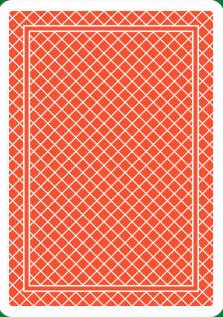

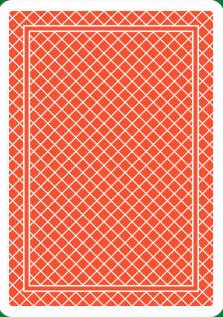

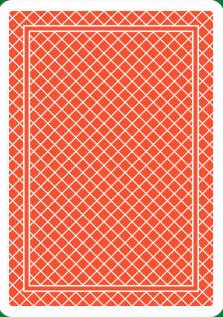

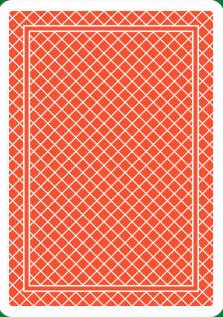

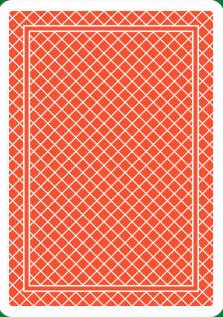

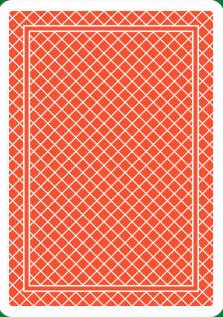

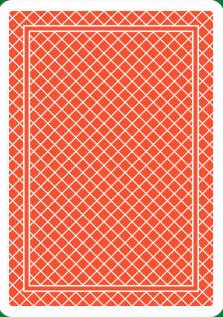

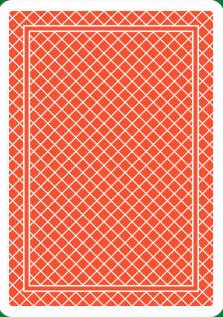

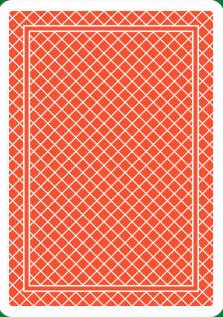

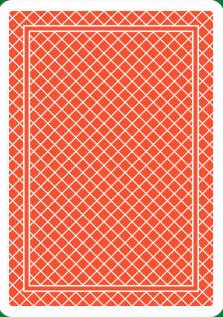

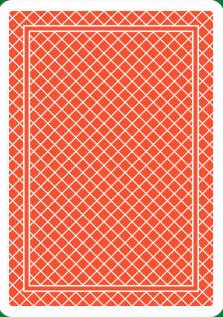

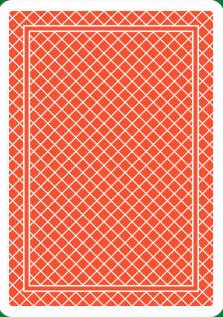

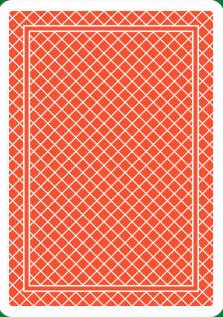
